Shell scripting in Linux is a pivotal aspect of Unix and Linux environments, offering a potent means to automate tasks. With it, users can execute a sequence of commands in a single script, significantly enhancing productivity. This comprehensive guide unravels the fundamental concepts of shell scripting, encompassing definitions, command syntax, and real-world examples for a profound grasp. #centlinux #linux #linuxcli
Table of Contents
Understanding Shell Scripting in Linux
What is Linux Shell Script?
Shell scripting in Linux is all about writing a series of commands in a text file to automate tasks on your Linux system. It’s like creating a recipe with instructions for your computer to follow, but instead of ingredients and cooking steps, you use Linux commands.
Here’s a breakdown of what shell scripting involves:
- Shell: The shell acts as an interpreter, reading the commands in your script and translating them into actions the computer can understand. Common Linux shells include Bash, Zsh, and Ksh.
- Scripts: These are plain text files containing the shell commands you want to execute. They typically end with the
.sh
extension. - Automating Tasks: The key benefit of shell scripting is automating repetitive tasks you might perform often on the command line. Imagine having a script to back up your files or update your system every week, saving you time and effort.
- Increased Efficiency: Scripting can streamline complex workflows by combining multiple commands into a single script. It also reduces the risk of errors compared to manually typing commands each time.
- Customization: Scripts can be tailored to your specific needs. You can include logic (like if/else statements) and loops to make your scripts more flexible and powerful.
Overall, shell scripting is a valuable tool for Linux users of all levels. It allows you to automate tasks, improve efficiency, and extend the functionality of your system without needing to be a programming expert.
Read Also: AWK Command: The Swiss Army Knife of Text Processing
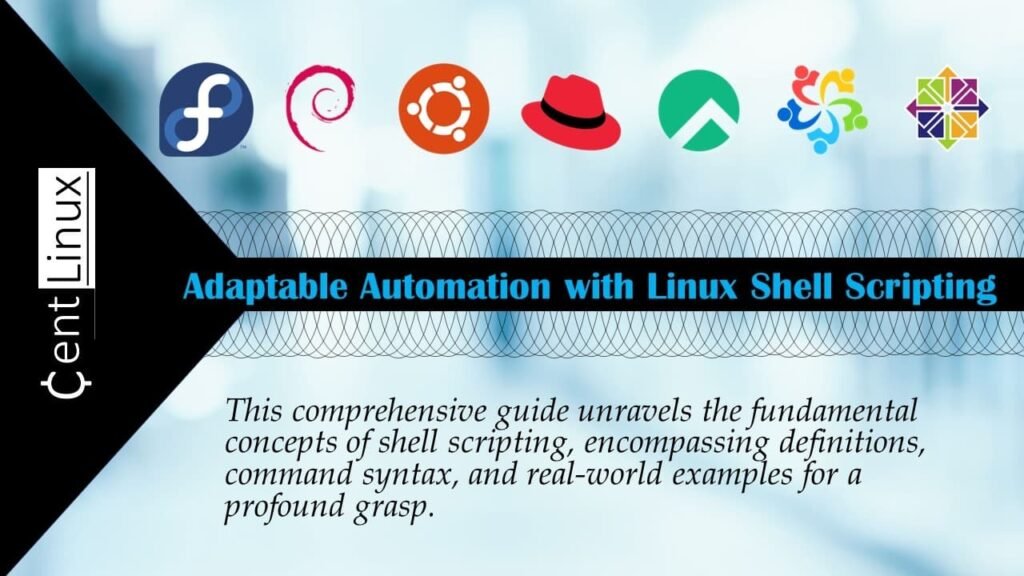
Core Terms used in Bash Scripting
Before diving into syntax and commands, let’s clarify some core terms used in Linux shell scripts:
- Shell: The interface through which a user interacts with the operating system.
- Script: A file containing a series of commands that can be executed.
- Shebang: The character sequence ‘#!’ that precedes the path to the shell interpreter.
- Variable: A symbol representing a value, allowing for dynamic data handling.
The Linux Memory Manager
$67.99 (as of June 30, 2025 20:24 GMT +00:00 – More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Basic Linux Shell Scripting Commands
The flexibility of Linux shell scripting is clearly demonstrated in its handling of variables, where explicit type declarations are not required. Unlike many programming languages that mandate predefined data types, shell scripting allows you to create and use variables dynamically without specifying whether they contain strings, numbers, or other data types. This simplicity not only makes shell scripting more accessible but also streamlines the process of writing scripts, enabling quick and efficient automation of tasks in a Linux environment.
// Variable Example
name="John Doe"
age=30
Conditions and Loops
Control structures, such as if
, else
, and loops like for
and while
, are fundamental components of programming that empower scripts to make decisions and perform repetitive tasks. The if
and else
statements allow you to evaluate conditions and execute specific blocks of code based on whether those conditions are met. Loops, such as for
and while
, provide the ability to iterate over a sequence of items or repeatedly execute a block of code until a specified condition is no longer true. Together, these control structures enhance the versatility and functionality of shell scripts, making it possible to automate complex workflows and adapt dynamically to different scenarios.
// If-Else Example
if [ condition ]; then
# commands
else
# commands
fi
Functions
Functions are an essential feature in programming, allowing you to group blocks of code into reusable units. By defining a function, you can encapsulate specific tasks or operations, making your scripts more modular, organized, and easier to maintain. For example, a function that adds two numbers can be reused multiple times within a script without the need to rewrite the same logic. This approach not only saves time but also ensures consistency and reduces the likelihood of errors. Functions enhance readability, promote efficient code management, and allow for scalability as your projects grow in complexity. Here’s an example of how a simple function can be designed to add two numbers and return the result.
// Function Example
function add_numbers {
sum=$(( $1 + $2 ))
echo "Sum: $sum"
}
add_numbers 5 3
300 Pcs Stickers for Kids, Cute Water Bottle Vinyl Waterproof Laptop Stickers for Students Gifts School Supplies Classroom Teacher Prizes Sticker Pack for Kids Girls Teens
$6.99 (as of June 30, 2025 20:05 GMT +00:00 – More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Input and Output
Shell scripts are highly versatile and can seamlessly interact with users by accepting input directly from the command line. This interaction is made possible through the use of the read
command, which prompts the user to provide data during the script’s execution. By incorporating user input, shell scripts can dynamically adapt their behavior, making them more interactive and user-friendly.
For instance, you can prompt the user for a filename, a number, or any other required value, and then process that input to perform specific actions. This capability is particularly useful for creating scripts that require user preferences or customization, enhancing the overall flexibility and usability of the script. By engaging users through prompts, you can ensure the script meets their specific needs and adapts to various scenarios.
// Input and Output Example
echo "Enter your name:"
read username
echo "Hello, $username!"
Practical Shell Scripting Examples
Automating Backups
The following is an example of a shell script designed to create a compressed backup of files, showcasing the power and efficiency of automation in Linux. This script allows users to specify the directory or files they wish to back up, the destination path, and the compression format (e.g., .tar.gz
, .zip
). By leveraging built-in Linux utilities like tar
or zip
, the script ensures that important data is archived and compressed, saving storage space and simplifying transfer or storage processes.
Such a script is invaluable for implementing regular backups, maintaining data integrity, and providing peace of mind in case of accidental deletions or system failures. Whether you’re managing personal files or a critical server, automating backups with a simple script ensures data is securely preserved with minimal manual intervention.
// Backup Script Example
#!/bin/bash
tar -czf backup.tar.gz /path/to/files
Razer Basilisk V3 Customizable Ergonomic Gaming Mouse: Fastest Gaming Mouse Switch – Chroma RGB Lighting – 26K DPI Optical Sensor – 11 Programmable Buttons – HyperScroll Tilt Wheel – Classic Black
$39.99 (as of June 29, 2025 23:03 GMT +00:00 – More infoProduct prices and availability are accurate as of the date/time indicated and are subject to change. Any price and availability information displayed on [relevant Amazon Site(s), as applicable] at the time of purchase will apply to the purchase of this product.)Batch Renaming Files
Imagine a scenario where you need to change the file extension for multiple files in a directory. For instance, you might have a collection of .txt
files that need to be converted to .csv
extensions for compatibility with another application. Manually renaming each file would be time-consuming and prone to errors, especially if there are many files involved.
This is where shell scripting becomes a powerful tool. By writing a script that loops through the directory and renames each file with the desired extension, you can automate the entire process. Such a script not only saves time but also ensures consistency and accuracy. This approach is particularly useful for developers, system administrators, or anyone handling bulk file operations regularly. Whether you’re preparing files for data analysis, archiving, or any other purpose, automating tasks like this demonstrates the efficiency and flexibility of Linux shell scripting.
// Batch Rename Example
#!/bin/bash
for file in *.txt; do
mv "$file" "${file%.txt}.doc"
done
Read Also: 10 Practical Tasks for RHCE Exam
Monitoring Disk Space
Here’s a practical example of a Linux script designed to monitor disk space usage and alert you when it exceeds a predefined threshold. Disk space management is a critical aspect of system administration, as insufficient disk space can lead to system crashes, application failures, or degraded performance.
This script periodically checks the usage of mounted filesystems and compares it to a specified threshold, such as 80% or 90%. If the usage exceeds this limit, the script generates an alert, which could be as simple as a terminal message or as advanced as an email or SMS notification. This kind of proactive monitoring ensures that you can address potential issues before they escalate, keeping your systems stable and your data safe.
Such scripts are invaluable for managing production servers, especially in environments with high data turnover or limited storage capacity. By automating disk usage checks, you save time, reduce manual intervention, and maintain an efficient, problem-free environment. This is just one example of how shell scripting can simplify and enhance routine system administration tasks.
// Disk Space Monitoring Example
#!/bin/bash
threshold=90
current=$(df -h | awk '//$/ {print $(NF-1)}' | tr -d '%')
if [ $current -gt $threshold ]; then
echo "Disk space exceeds $threshold%."
fi
Recommended Training: The Linux Command Line Bootcamp: Beginner To Power User from Colt Steele

Final Thoughts
Shell scripting in Linux is an essential skill for system administrators, developers, and IT professionals working in Unix or Linux environments. It serves as a powerful tool for automating repetitive tasks, managing system processes, and simplifying complex workflows. From automating backups and monitoring system health to deploying applications and configuring servers, shell scripting plays a pivotal role in streamlining system management.
By mastering the syntax, understanding control structures, and becoming proficient with Linux commands, you can write custom scripts tailored to your specific needs. These scripts not only save time but also reduce the risk of human error, increase efficiency, and ensure consistency in task execution. Shell scripting empowers you to take full control of your environment, making it an indispensable skill for boosting workflow and productivity in today’s fast-paced tech landscape.
Your Linux servers deserve expert care! I provide reliable management and optimization services tailored to your needs. Discover how I can help on Fiverr!
Leave a Reply
You must be logged in to post a comment.